How to Create a JSON File in Java: Beginner to Advanced Guide
Imad Uddin
Full Stack Developer
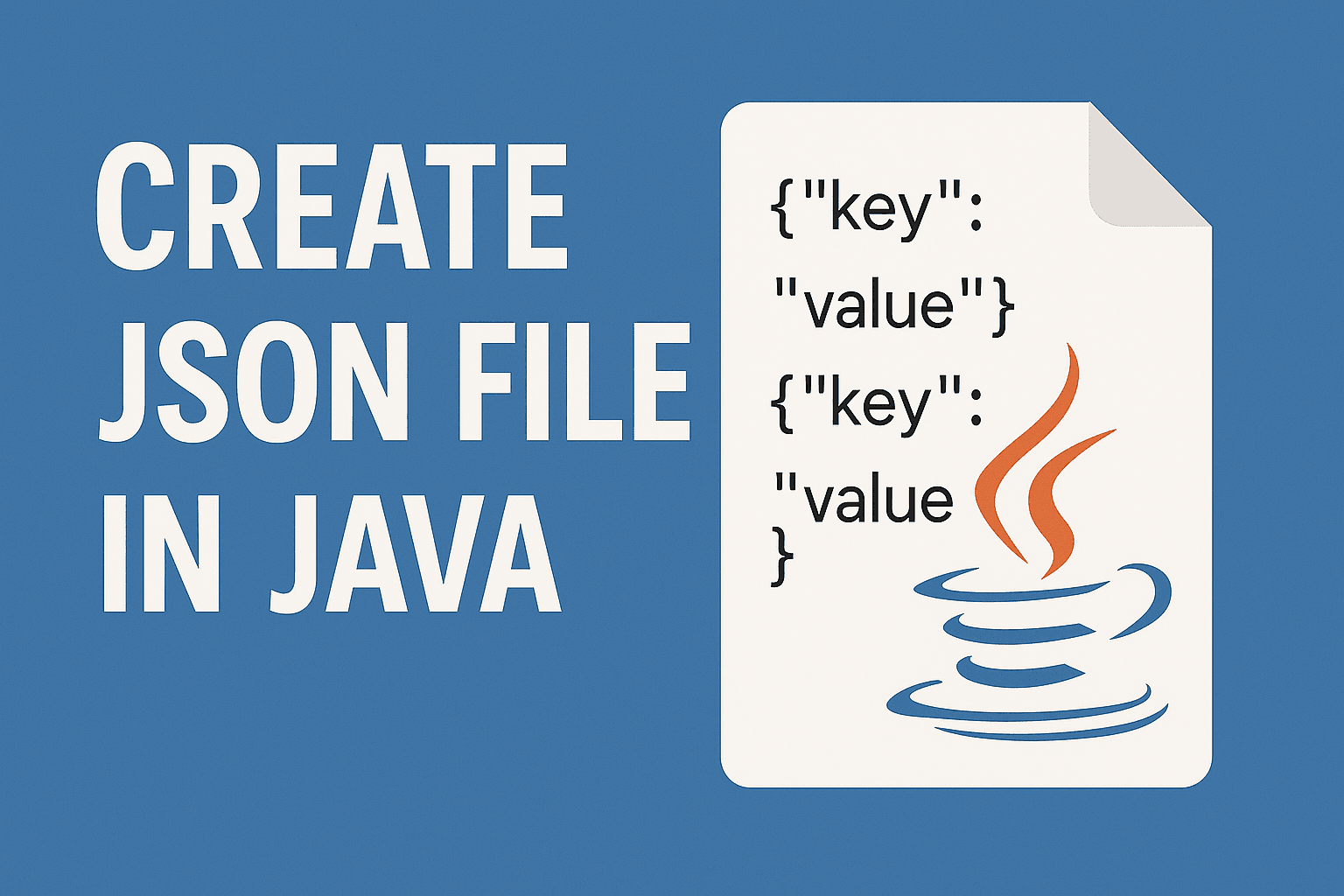
JSON (JavaScript Object Notation) is a versatile, lightweight data format integral to modern software development. For Java developers, learning to create JSON files in Java is essential for tasks like data storage, API integration, and configuration management. If you're new to Java and searching for a clear guide on how to create a JSON file in Java, this comprehensive, beginner-friendly tutorial is designed for you. I have tried my level best to make sure it offers a professional, step-by-step approach using three popular libraries—Jackson, Gson, and JSON-P—crafted to empower begginers with practical skills.
This guide covers:
- An introduction to JSON and its significance in Java
- Setting up a Java development environment
- Detailed instructions for creating JSON files with three libraries
- Practical, copy-paste code examples and real-world use cases
- Common errors and troubleshooting strategies
- Frequently asked questions to address beginner queries
Whether you're building a simple project or more advanced applications, this article helps you to create JSON files with confidence. Let’s begin.
Understanding JSON: A Beginner’s Overview 🌟
JSON is a text-based format for structuring data, akin to a well-organized digital notebook. It uses key-value pairs to represent information, grouped within objects (enclosed in curly braces {}) or arrays (enclosed in square brackets []). Here’s a sample JSON object:
Code Snippet{ "name": "Alice", "age": 20, "isStudent": true, "hobbies": ["reading", "coding"] }
This example describes a student named Alice, with attributes like age and hobbies. JSON’s readability and compatibility make it ideal for data exchange across web APIs, mobile apps, and more.
In Java, creating a JSON file involves programmatically generating such structured data and saving it as a .json file. This skill is foundational for handling data in real-world applications.
Why Create JSON Files in Java? 🤔
Java’s reliability and scalability make it a cornerstone of enterprise systems, Android development, and backend services. Creating JSON files in Java enables:
- Data Storage: Persist application data, such as user profiles or settings.
- API Communication: Format data for seamless interaction with web services.
- Configuration Files: Define application settings in a portable, human-readable format.
For beginners, learning to create JSON files builds a strong foundation for advanced Java projects and data-driven development.
Setting Up Your Java Development Environment 🛠️
Before coding, you need a properly configured Java environment. This section provides clear instructions to ensure even complete novices can set up successfully.
Installing the Java Development Kit (JDK)
The JDK is essential for compiling and running Java programs. Follow these steps:
- Download the latest JDK (e.g., JDK 17) from the Oracle JDK website or OpenJDK.
- Install the JDK, adhering to the instructions for your operating system (Windows, macOS, or Linux).
- Verify the installation by opening a terminal or command prompt and running:
Code Snippetjava -version
You should see output like java version "17.0.8". If errors occur, confirm the JDK is installed and added to your system’s PATH environment variable.
Choosing an Integrated Development Environment (IDE)
An IDE enhances coding efficiency with features like code completion and debugging. Recommended IDEs for beginners include:
- IntelliJ IDEA Community Edition: Free, user-friendly, and feature-rich.
- Eclipse: Free, widely adopted in Java development.
- Visual Studio Code: Lightweight, with Java extensions for simplicity.
Download and install your preferred IDE to proceed.
Creating a Java Project
Initialize a new project in your IDE:
- Open your IDE and select “New Project.”
- Choose “Java” as the project type and select your installed JDK.
- Name the project (e.g., JsonTutorial) and create it.
Your environment is now ready for JSON development.
Libraries for JSON Processing in Java 📚
Java does not natively support JSON, but libraries simplify the process of creating JSON files. This guide focuses on three widely used libraries:
- Jackson: A high-performance library for complex JSON tasks.
- Gson: A lightweight, beginner-friendly library developed by Google.
- JSON-P (javax.json): A standard Java EE API for structured JSON processing.
These libraries streamline JSON creation, ensuring accuracy and efficiency. We’ll explore each with detailed, beginner-friendly examples.
Method 1: Creating a JSON File with Jackson 🚀
Jackson is a robust, high-performance library favored for its versatility, making it suitable for both beginners and advanced developers.
Adding Jackson to Your Project
If using Maven, add the Jackson dependency to your pom.xml:
Code Snippet<dependencies> <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-databind</artifactId> <version>2.15.2</version> </dependency> </dependencies>
For non-Maven projects, download Jackson JARs from Maven Central and include them in your IDE’s classpath.
Writing Code to Create a JSON File
Create a Java class named CreateJsonWithJackson.java and use the following code to generate a JSON file representing a student:
Code Snippetimport com.fasterxml.jackson.databind.ObjectMapper; import java.io.File; import java.util.Arrays; import java.util.HashMap; import java.util.Map; public class CreateJsonWithJackson { public static void main(String[] args) { try { // Initialize a Map to store JSON data Map<String, Object> student = new HashMap<>(); student.put("name", "Alice"); student.put("age", 20); student.put("isStudent", true); student.put("hobbies", Arrays.asList("reading", "coding")); // Instantiate Jackson's ObjectMapper ObjectMapper mapper = new ObjectMapper(); // Write JSON data to a file mapper.writeValue(new File("student.json"), student); System.out.println("JSON file created successfully!"); } catch (Exception e) { System.out.println("Error creating JSON file: " + e.getMessage()); } } }
Running the Code
- Execute the program in your IDE (typically via a “Run” button or shortcut).
- Locate student.json in your project’s root directory.
- Open the file in a text editor to verify its contents:
Code Snippet{ "name": "Alice", "age": 20, "isStudent": true, "hobbies": ["reading", "coding"] }
How Jackson Works
- Map: A HashMap stores key-value pairs, mirroring JSON’s structure.
- ObjectMapper: Jackson’s core class converts Java objects to JSON.
- writeValue: Serializes the Map to a JSON file.
Jackson’s efficiency and support for complex data structures make it a preferred choice for professional Java applications.
Method 2: Creating a JSON File with Gson 🌈
Gson, developed by Google, offers a simple, intuitive API, making it an excellent choice for beginners learning to write JSON files in Java.
Adding Gson to Your Project
For Maven, include this dependency in pom.xml:
Code Snippet<dependencies> <dependency> <groupId>com.google.code.gson</groupId> <artifactId>gson</artifactId> <version>2.10.1</version> </dependency> </dependencies>
Alternatively, download Gson JARs from Maven Central and add them to your project.
Writing Code to Create a JSON File
Create a class named CreateJsonWithGson.java with the following code:
Code Snippetimport com.google.gson.Gson; import com.google.gson.GsonBuilder; import java.io.FileWriter; import java.util.Arrays; import java.util.HashMap; import java.util.Map; public class CreateJsonWithGson { public static void main(String[] args) { try { // Create a Map to hold JSON data Map<String, Object> student = new HashMap<>(); student.put("name", "Bob"); student.put("age", 22); student.put("isStudent", true); student.put("hobbies", Arrays.asList("gaming", "music")); // Configure Gson for formatted output Gson gson = new GsonBuilder().setPrettyPrinting().create(); // Write JSON to a file FileWriter writer = new FileWriter("student_gson.json"); gson.toJson(student, writer); writer.close(); System.out.println("JSON file created successfully!"); } catch (Exception e) { System.out.println("Error creating JSON file: " + e.getMessage()); } } }
Running the Code
Run the program and check for student_gson.json in your project directory. The file should contain:
Code Snippet{ "name": "Bob", "age": 22, "isStudent": true, "hobbies": ["gaming", "music"] }
How Gson Works
- GsonBuilder: Enables pretty printing for readable JSON output.
- toJson: Serializes the Map to JSON, writing it via FileWriter.
- FileWriter: Manages file output operations.
Gson’s straightforward API makes it ideal for beginners and small-scale projects.
Method 3: Creating a JSON File with JSON-P (javax.json) 🧩
JSON-P, part of Java EE, provides a structured, programmatic approach to JSON processing, offering insight into JSON’s mechanics.
Adding JSON-P to Your Project
For Maven, add these dependencies to pom.xml:
Code Snippet<dependencies> <dependency> <groupId>javax.json</groupId> <artifactId>javax.json-api</artifactId> <version>1.1.4</version> </dependency> <dependency> <groupId>org.glassfish</groupId> <artifactId>javax.json</artifactId> <version>1.1.4</version> </dependency> </dependencies>
For non-Maven setups, download JARs from Maven Central.
Writing Code to Create a JSON File
Create a class named CreateJsonWithJsonP.java:
Code Snippetimport javax.json.*; import java.io.FileWriter; public class CreateJsonWithJsonP { public static void main(String[] args) { try { // Build a JSON object JsonObject student = Json.createObjectBuilder() .add("name", "Charlie") .add("age", 21) .add("isStudent", true) .add("hobbies", Json.createArrayBuilder() .add("painting") .add("hiking") .build()) .build(); // Write JSON to a file FileWriter writer = new FileWriter("student_jsonp.json"); JsonWriter jsonWriter = Json.createWriter(writer); jsonWriter.writeObject(student); jsonWriter.close(); System.out.println("JSON file created successfully!"); } catch (Exception e) { System.out.println("Error creating JSON file: " + e.getMessage()); } } }
Running the Code
Execute the program and verify student_jsonp.json:
Code Snippet{ "name": "Charlie", "age": 21, "isStudent": true, "hobbies": ["painting", "hiking"] }
How JSON-P Works
- JsonObjectBuilder: Constructs JSON objects incrementally.
- JsonWriter: Outputs the JSON structure to a file.
- createArrayBuilder: Manages JSON arrays.
JSON-P’s explicit approach is educational but less common in modern applications compared to Jackson or Gson.
Comparing Jackson, Gson, and JSON-P 📊
To choose the right library, consider this comparison:
Library | Ease of Use | Performance | Features | Ideal Use Case |
---|---|---|---|---|
Jackson | Moderate | High | Advanced (e.g., annotations) | Complex, enterprise projects |
Gson | Very High | Moderate | Simple API | Beginners, small projects |
JSON-P | Moderate | Lower | Standard API | Java EE, educational purposes |
For beginners, Gson offers the simplest entry point, while Jackson prepares you for professional environments.
Advanced Example: Building a Complex JSON File 📖
To demonstrate real-world applications, let’s create a JSON file for a library catalog using Jackson, showcasing nested objects and arrays.
Create a class named CreateComplexJson.java:
Code Snippetimport com.fasterxml.jackson.databind.ObjectMapper; import java.io.File; import java.util.Arrays; import java.util.HashMap; import java.util.Map; public class CreateComplexJson { public static void main(String[] args) { try { // Define book 1 Map<String, Object> book1 = new HashMap<>(); book1.put("title", "The Hobbit"); book1.put("author", "J.R.R. Tolkien"); book1.put("year", 1937); book1.put("genres", Arrays.asList("Fantasy", "Adventure")); // Define book 2 Map<String, Object> book2 = new HashMap<>(); book2.put("title", "1984"); book2.put("author", "George Orwell"); book2.put("year", 1949); book2.put("genres", Arrays.asList("Dystopian", "Fiction")); // Create library structure Map<String, Object> library = new HashMap<>(); library.put("libraryName", "City Library"); library.put("books", Arrays.asList(book1, book2)); // Write to JSON file ObjectMapper mapper = new ObjectMapper(); mapper.writeValue(new File("library.json"), library); System.out.println("Complex JSON file created successfully!"); } catch (Exception e) { System.out.println("Error creating JSON file: " + e.getMessage()); } } }
This generates library.json:
Code Snippet{ "libraryName": "City Library", "books": [ { "title": "The Hobbit", "author": "J.R.R. Tolkien", "year": 1937, "genres": ["Fantasy", "Adventure"] }, { "title": "1984", "author": "George Orwell", "year": 1949, "genres": ["Dystopian", "Fiction"] } ] }
This example illustrates hierarchical JSON structures, common in applications like inventory systems or APIs.
Troubleshooting Common Issues 🛡️
Beginners may encounter challenges when creating JSON files. Here are solutions to common problems:
-
File Not Found Exception
- Cause: Incorrect file path or lack of write permissions.
- Solution: Use a relative path like new File("student.json") to save in the project directory. Ensure write access to the target folder.
-
Class Not Found Error
- Cause: Missing library dependencies.
- Solution: Confirm JARs are included in the classpath. For Maven, run mvn clean install.
-
Invalid JSON Syntax
- Cause: Errors in manual JSON construction.
- Solution: Rely on libraries to handle formatting. Validate output using tools like JSONLint.
-
Permission Denied
- Cause: Restricted file system access.
- Solution: Run your IDE with administrative privileges or save files to an accessible directory, such as your project folder.
Best Practices for Beginners 🌱
To optimize your JSON creation workflow:
- Start with Simple Structures: Begin with basic key-value pairs before exploring nested objects.
- Use Pretty Printing: Leverage library features to format JSON for readability.
- Validate Output: Use online validators to ensure JSON correctness.
- Expand Your Skills: After mastering creation, explore JSON parsing or API integration.
Frequently Asked Questions ❓
What is the simplest method to create a JSON file in Java?
Gson is the most beginner-friendly due to its intuitive API. Refer to the Gson example above for a straightforward implementation.
Are libraries necessary for JSON creation in Java?
While manual string manipulation is possible, libraries like Jackson, Gson, and JSON-P prevent errors and streamline the process, making them highly recommended.
Can I create JSON files without an IDE?
Yes, you can write and compile Java code using a text editor and the command line. However, IDEs like IntelliJ IDEA enhance productivity with debugging and code completion.
How do I ensure my JSON file is formatted correctly?
Use pretty printing features, such as Gson’s setPrettyPrinting() or Jackson’s writerWithDefaultPrettyPrinter(), to produce readable, indented JSON.
How does Jackson compare to Gson for JSON creation?
Jackson offers superior performance and advanced features, ideal for large projects. Gson prioritizes simplicity, making it better for beginners. See the comparison table for details.
Conclusion 🎉
Mastering the art of creating JSON files in Java is a critical skill for developers, enabling data-driven applications and API integrations. In this guide I have provided a comprehensive, beginner-friendly roadmap to using Jackson, Gson, and JSON-P, supported by practical examples and troubleshooting advice. Whether you’re starting your Java journey or advancing your skills, these techniques will empower your projects.
Experiment with the provided code, adapt it to your needs, and explore related topics like parsing JSON in Java or validating JSON schemas. Share this guide if you find it helpful. For further resources, visit Maven Central or validate your JSON with JSONLint.